Best Practices for Embedded Software Development
- rashidjavedrj07
- 2 days ago
- 13 min read
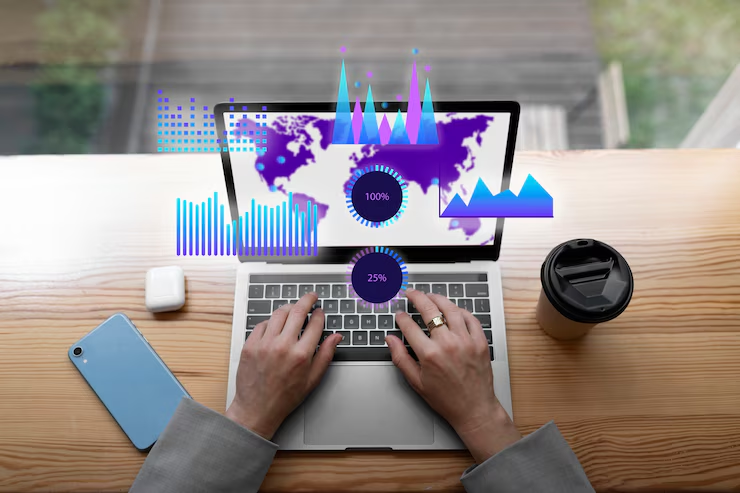
Before diving into best practices, it's essential to understand what embedded software is. Embedded software is a type of software designed to control machines or devices that are not traditional computers. These can range from small devices like a digital watch to larger systems like a car's engine management system.
The Role of Embedded Software
Embedded software is integral to the functioning of the device it controls. It needs to be highly reliable and efficient due to the limited resources available on embedded systems, such as memory and processing power. The software often acts as the brain of the device, interpreting inputs and controlling outputs to achieve the desired functionality.
Differences from Traditional Software
Unlike traditional software, which runs on general-purpose computers, embedded software runs on dedicated hardware. This means it often interacts directly with hardware components, requiring a deep understanding of the underlying hardware architecture. Additionally, embedded software development often involves working with constraints such as limited power supply, which affects design choices.
Applications of Embedded Software
Embedded software is everywhere, from household appliances like microwaves and washing machines to critical systems like medical devices and industrial machines. Each application has unique requirements and constraints, which makes flexibility and adaptability crucial skills for embedded software developers. Understanding these varied applications helps developers appreciate the breadth and impact of their work.
Key Considerations in Embedded Software Development
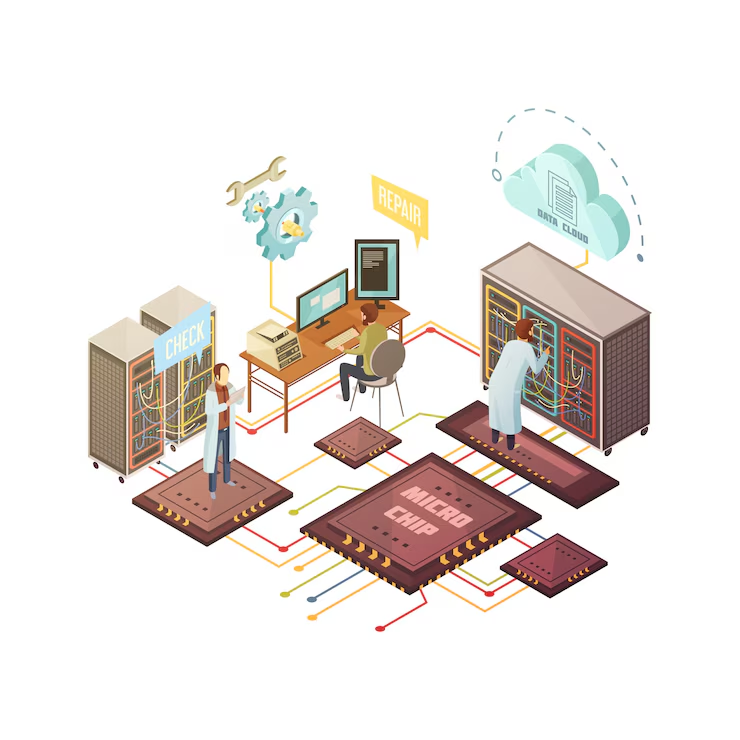
Resource Constraints
Embedded systems usually operate with limited computing resources. This means that the software must be optimized for speed and memory usage. Developers need to be mindful of these constraints when designing and coding.
Memory Management
Memory is a precious resource in embedded systems, and efficient memory management is crucial. Developers must ensure that memory is allocated and deallocated properly to prevent leaks, which can cause system crashes. Techniques such as static memory allocation and careful stack usage are often employed to manage memory efficiently.
Processing Power
Limited processing power requires developers to write highly optimized code. This involves choosing efficient algorithms and data structures that make the best use of CPU cycles. Developers often need to balance performance with power consumption, especially in battery-operated devices, to extend the device's operational life.
Power Consumption
Power consumption is a critical consideration, particularly for portable and battery-powered devices. Developers must design software that minimizes power usage, which can involve reducing CPU activity, optimizing peripheral usage, and implementing power-saving modes. Understanding the power profile of an application is essential for achieving energy efficiency.
Real-Time Requirements
Many embedded systems are real-time systems, meaning they need to respond to inputs or changes in the environment within a specific time frame. Failing to do so can lead to system failures or unsafe conditions. Real-time requirements should be considered from the start of the development process.
Understanding Real-Time Constraints
Real-time constraints dictate that the software must complete its tasks within a predetermined time. This requires careful scheduling and prioritization of tasks to ensure that critical operations are performed on time. Developers use techniques like preemptive multitasking and interrupt handling to manage real-time constraints effectively.
Implementing Real-Time Solutions
Implementing real-time solutions often involves using a Real-Time Operating System (RTOS) that provides the necessary scheduling and resource management capabilities. An RTOS helps manage task priorities and ensures that deadlines are consistently met. Choosing the right RTOS and configuring it correctly is crucial for the success of real-time applications.
Testing Real-Time Systems
Testing real-time systems is complex due to the need to validate timing constraints. Developers use specialized testing tools and techniques to simulate real-world conditions and verify that the system meets its real-time requirements. Timing analysis and stress testing are integral parts of ensuring that real-time systems perform reliably under all conditions.
Best Practices
1. Start with a Clear Specification
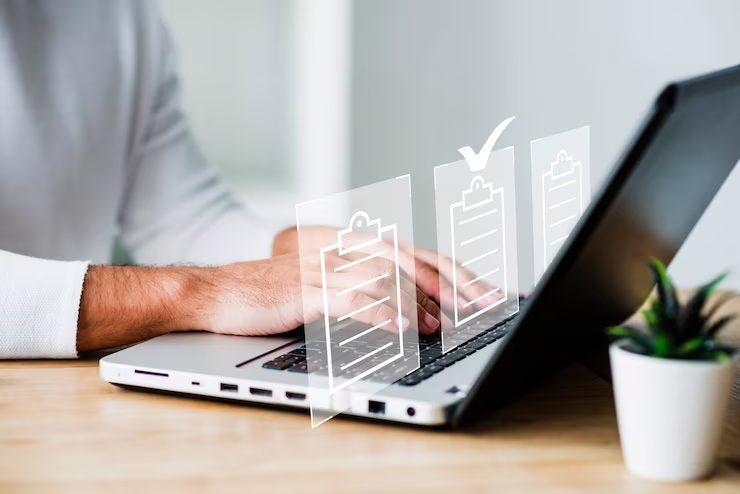
Before you begin coding, it's crucial to have a clear understanding of what the software needs to do. Create a detailed specification that includes all functional and non-functional requirements. This document will serve as a roadmap throughout the development process.
Importance of Detailed Specifications
Detailed specifications provide a blueprint for the development process, ensuring that all team members have a shared understanding of the project goals. Specifications should include precise descriptions of what the software must accomplish, as well as constraints and performance metrics. This clarity helps prevent scope creep and ensures that the final product meets user expectations.
Developing Functional Requirements
Functional requirements specify what the software should do, detailing inputs, outputs, and behavior under various conditions. They form the core of the specification document, guiding the design and implementation phases. Developers should work closely with stakeholders to capture all necessary functionality accurately.
Non-Functional Requirements and Constraints
Non-functional requirements encompass performance, usability, reliability, and other quality attributes. These requirements are crucial for embedded systems, which often operate in constrained environments. Defining non-functional requirements early helps prioritize design decisions and ensures that the software meets critical quality standards.
2. Use Modular Design
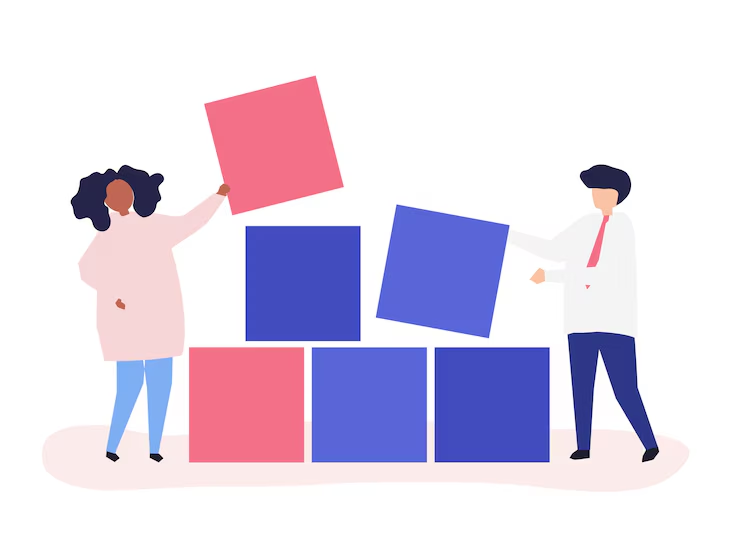
Modular design involves breaking down the software into smaller, manageable pieces or modules. This approach makes the software easier to understand, test, and maintain. Each module should have a single responsibility and be as independent as possible from other modules.
Benefits of Modularity
Modularity enhances flexibility and scalability by allowing developers to work on different parts of the software simultaneously without interference. It also simplifies debugging and testing since individual modules can be isolated and tested independently. This separation of concerns reduces complexity and leads to more maintainable and robust code.
Designing Independent Modules
Each module should perform a specific function and communicate with other modules through well-defined interfaces. Developers should aim to minimize dependencies between modules to enhance reusability and ease of maintenance. Clear interface definitions ensure that changes within a module don't affect others, supporting long-term code stability.
Testing and Maintaining Modules
Testing modular systems is more straightforward because each module can be tested in isolation before integrating it into the larger system. This approach helps identify defects early and simplifies troubleshooting. Regular maintenance is also easier, as updates or bug fixes can be applied to individual modules without impacting the entire system.
3. Optimize Code for Performance
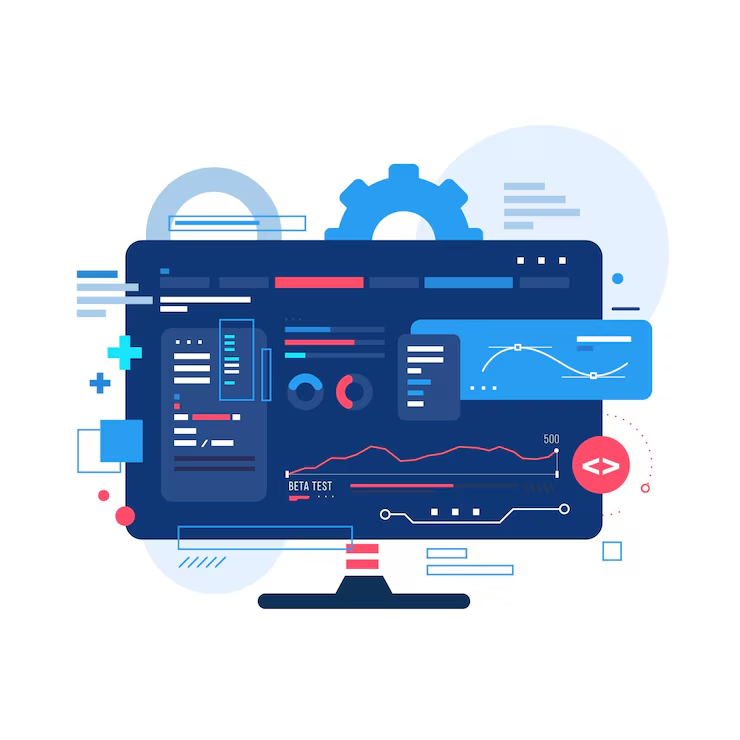
Due to the limited resources in embedded systems, optimizing code is a necessity. This includes writing efficient algorithms and avoiding unnecessary computations. Use profiling tools to identify and optimize performance bottlenecks.
Writing Efficient Algorithms
Selecting the right algorithms is crucial for performance optimization. Developers should focus on algorithms with optimal time and space complexity, balancing these factors against the system's constraints. Implementing custom-tailored solutions for specific hardware can also yield significant performance gains.
Minimizing Unnecessary Computations
Reducing unnecessary computations involves simplifying code logic and eliminating redundant operations. Loop unrolling, inlining functions, and optimizing data access patterns are techniques that can lead to more efficient code. Developers must carefully analyze the trade-offs of each optimization to ensure they don't introduce new issues.
Using Profiling Tools
Profiling tools help developers identify performance bottlenecks by providing insights into the software's runtime behavior. By analyzing execution time, memory usage, and CPU load, developers can target specific areas for optimization. Regular profiling throughout the development process helps maintain performance standards and prevent regressions.
4. Implement Robust Testing

Testing is crucial in embedded software development. It helps ensure that the software behaves as expected and meets all requirements. Use a combination of unit testing, integration testing, and system testing. Simulators and emulators can also be helpful for testing in an environment similar to the target hardware.
Unit Testing and Its Importance
Unit testing involves testing individual components or functions in isolation to ensure they work correctly. This practice helps catch errors early in the development process, reducing the likelihood of bugs in the final product. Automated unit tests provide a safety net for developers, enabling quick detection of regressions during code changes.
Integration and System Testing
Integration testing focuses on ensuring that different modules work together as expected. It involves testing the interfaces and interactions between components to uncover issues that unit tests might miss. System testing evaluates the entire software application in a real or simulated environment, verifying that it meets both functional and non-functional requirements.
Utilizing Simulators and Emulators
Simulators and emulators provide a controlled environment for testing embedded software without needing physical hardware. They allow developers to test scenarios that might be difficult or expensive to replicate in real-world conditions. These tools are invaluable for verifying software behavior and identifying issues early, especially in the absence of complete hardware setups.
5. Prioritize Reliability and Safety
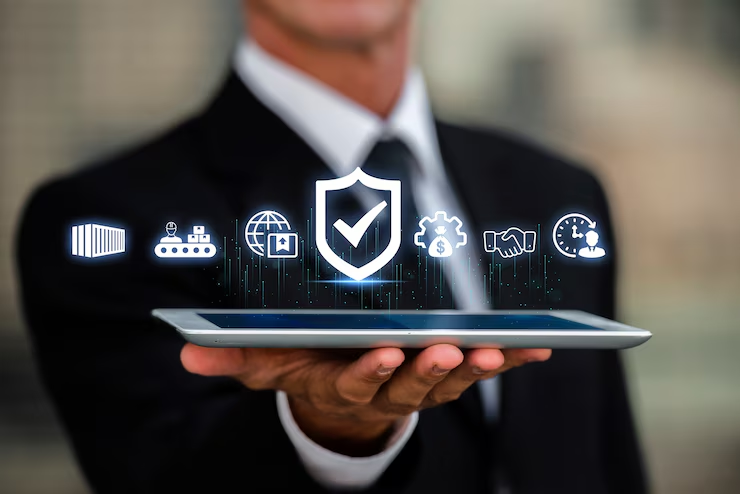
In many cases, embedded software is used in critical systems where failures can lead to severe consequences. Prioritize reliability and safety by implementing error handling and recovery strategies. Consider using formal methods to prove the correctness of critical components.
Implementing Error Handling
Robust error handling involves anticipating potential faults and implementing strategies to mitigate their impact. Developers should design software that gracefully recovers from errors, maintaining critical functionality and ensuring user safety. Logging and monitoring systems can provide valuable insights into errors and aid in troubleshooting.
Ensuring System Safety
System safety is paramount in applications where software failures could lead to catastrophic outcomes. Developers should conduct thorough risk assessments and implement fail-safe mechanisms to prevent unsafe conditions. Safety-critical systems often require adherence to industry standards and rigorous testing to ensure compliance.
Using Formal Methods
Formal methods involve mathematically proving the correctness of software algorithms and logic. These techniques provide a high level of assurance for critical components, reducing the likelihood of defects. While formal methods can be complex and resource-intensive, they are invaluable for applications where safety and reliability are non-negotiable.
6. Use Version Control Systems
Version control systems like Git help manage changes to the software code over time. They allow teams to collaborate efficiently, track changes, and revert to previous versions if needed. Using version control is a standard practice in software engineering that should not be overlooked.
Benefits of Version Control
Version control systems provide a history of code changes, enabling developers to track modifications and understand how the software has evolved. This history is crucial for diagnosing issues and rolling back changes that introduce bugs. Version control also facilitates collaboration by allowing multiple developers to work on different branches simultaneously.
Implementing Effective Branching Strategies
Branching strategies help manage development workflows, ensuring that changes are organized and integrated smoothly. Common strategies include feature branches, release branches, and hotfix branches. By maintaining a structured approach to branching, teams can manage parallel development efforts and streamline the release process.
Leveraging Version Control for Collaboration
Version control systems enable seamless collaboration among team members, even when working remotely. They provide tools for code review, merge conflict resolution, and collaborative decision-making. Effective use of version control fosters a culture of transparency and accountability within development teams.
7. Regularly Review and Refactor Code
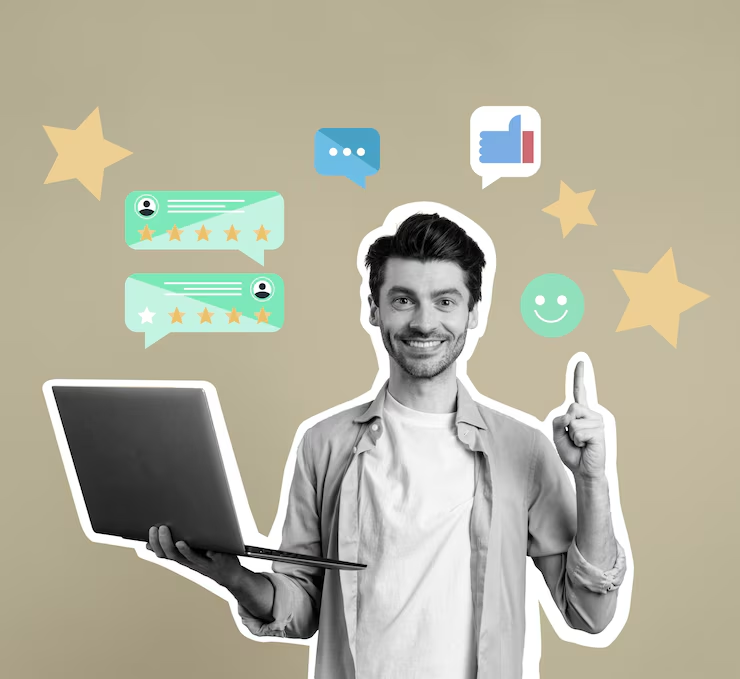
Code reviews are a great way to catch potential issues early and improve the quality of the software. Encourage team members to participate in peer reviews and be open to feedback. Regularly refactor code to improve its structure and readability without altering its functionality.
Conducting Effective Code Reviews
Code reviews involve examining code changes to identify defects, ensure adherence to coding standards, and provide constructive feedback. They foster knowledge sharing and improve code quality by incorporating diverse perspectives. Encouraging a positive review culture helps team members grow and contributes to the overall success of the project.
Benefits of Code Refactoring
Refactoring involves restructuring existing code to improve its readability, maintainability, and performance. It eliminates technical debt and keeps the codebase clean, which is essential for long-term project success. Regular refactoring ensures that the software remains flexible and adaptable to new requirements.
Encouraging a Culture of Continuous Improvement
A culture of continuous improvement emphasizes learning, growth, and adaptation. By regularly reviewing and refactoring code, teams can incrementally enhance their processes and products. Encouraging open communication and feedback helps identify areas for improvement and fosters innovation.
8. Document Thoroughly
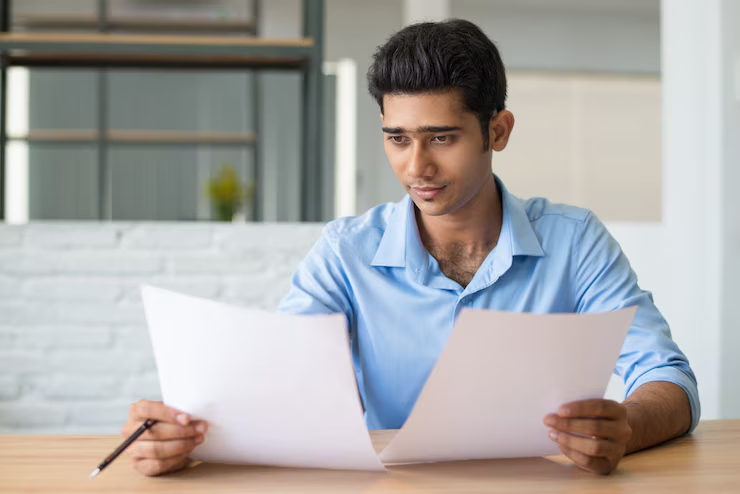
Documentation is often overlooked but is essential for the long-term success of any software project. Keep documentation up-to-date and include details about the design, architecture, and usage of the software. This will be invaluable for future maintenance and onboarding new team members.
Creating Comprehensive Documentation
Comprehensive documentation includes user manuals, design documents, API references, and code comments. It provides a detailed understanding of the software's functionality, architecture, and use cases. Well-documented projects facilitate knowledge transfer and enable new team members to get up to speed quickly.
Maintaining Up-to-Date Documentation
Keeping documentation current is crucial as software evolves. Regular updates ensure that documentation accurately reflects the software's state and guides users and developers effectively. Establishing documentation as a continuous process, rather than a one-time task, helps maintain its relevance and utility.
Leveraging Documentation for Knowledge Sharing
Documentation serves as a valuable knowledge repository, capturing insights and decisions made during the development process. It supports collaboration by providing a common reference point for team members. By investing in thorough documentation, teams can preserve institutional knowledge and enhance their collective expertise.
Tools and Techniques
Integrated Development Environments (IDEs)
IDEs provide a comprehensive environment for software development. They offer features like code editing, debugging, and project management. Choose an IDE that supports the language and platform you are working with.
Features of Modern IDEs
Modern IDEs are equipped with advanced features such as syntax highlighting, code autocompletion, and integrated debugging tools. These features enhance productivity by simplifying common development tasks and reducing the likelihood of errors. IDEs also often include project management tools that help organize files, manage dependencies, and streamline the build process.
Choosing the Right IDE
Selecting the right IDE depends on the project's requirements, the programming language used, and the target platform. Developers should consider factors such as ease of use, community support, and available plugins when choosing an IDE. Familiarity with the IDE can significantly impact development efficiency and project success.
Customizing and Extending IDEs
Many IDEs offer customization options that allow developers to tailor the environment to their workflow. Plugins and extensions can add new features, integrate with version control systems, or support additional programming languages. Customizing the IDE enhances productivity by aligning the development environment with individual preferences and project needs.
Debugging Tools
Embedded systems can be challenging to debug due to their constrained environments. Use debugging tools that allow you to inspect the state of the system and trace the execution of code. Hardware debuggers can be particularly useful for embedded systems.
The Role of Debugging Tools
Debugging tools help developers identify and resolve issues by providing insights into the software's execution. They allow developers to set breakpoints, inspect variables, and step through code to understand its behavior. Effective debugging is essential for diagnosing and fixing defects, especially in complex embedded systems.
Using Software Debuggers
Software debuggers are integrated into IDEs and provide a range of features for examining code execution in real-time. They offer a high-level view of the software's behavior, making them suitable for catching logical errors and understanding program flow. Software debuggers are invaluable for initial testing and debugging phases.
Leveraging Hardware Debuggers
Hardware debuggers provide low-level access to the embedded system, enabling developers to inspect the state of the hardware directly. They are essential for diagnosing hardware-software integration issues and ensuring that the software interacts correctly with the hardware. Hardware debuggers are especially useful for real-time systems and applications with stringent timing constraints.
Real-Time Operating Systems (RTOS)
In systems with real-time requirements, an RTOS can help manage tasks and ensure that deadlines are met. An RTOS provides scheduling, resource management, and other services that are crucial for real-time applications.
Advantages of Using an RTOS
An RTOS offers deterministic task scheduling, which is critical for meeting real-time constraints. It provides mechanisms for managing concurrency, resource allocation, and inter-process communication, simplifying the development of complex systems. By abstracting hardware details, an RTOS allows developers to focus on application logic and reduce development time.
Selecting the Right RTOS
Choosing the appropriate RTOS involves evaluating factors such as licensing, support, and compatibility with the target hardware. Developers should consider the RTOS's feature set, ease of use, and community support when making a selection. The chosen RTOS should align with the system's requirements and facilitate efficient implementation of real-time functionality.
Configuring and Using an RTOS
Proper configuration of an RTOS is essential for optimizing system performance and meeting real-time constraints. Developers must configure task priorities, scheduling policies, and resource management settings to suit the application's needs. Understanding the RTOS's APIs and services is crucial for leveraging its full potential and ensuring system reliability.
Challenges in Embedded Software Development
Hardware Limitations
One of the primary challenges in embedded software development is dealing with hardware limitations. Developers must work within the constraints of the hardware, which can include limited memory, processing power, and input/output capabilities.
Adapting to Hardware Constraints
Developers need to tailor their software to fit within the hardware's constraints, which often requires innovative solutions and compromises. This might involve optimizing code to reduce memory usage or finding ways to perform computations more efficiently. A deep understanding of the hardware's capabilities is crucial for making informed design decisions.
Balancing Performance and Resource Usage
Balancing performance with resource usage is a constant challenge in embedded systems. Developers must prioritize critical functionality while minimizing resource consumption to achieve optimal performance. This balance often requires iterative testing and refinement to ensure the software operates effectively within the hardware's limits.
Leveraging Hardware Features
Utilizing hardware-specific features can enhance software performance and functionality. Many embedded systems offer specialized hardware accelerators or peripheral interfaces that developers can exploit to offload processing tasks. Leveraging these features requires thorough knowledge of the hardware architecture and capabilities.
Integration with Hardware
Ensuring that the software integrates seamlessly with the hardware is another challenge. This requires a good understanding of the hardware specifications and often involves close collaboration with hardware engineers.
Understanding Hardware Specifications
Developers must thoroughly understand the hardware specifications to ensure successful integration. This includes knowledge of communication protocols, memory maps, and peripheral interfaces. Familiarity with datasheets and technical documentation is essential for making informed integration decisions.
Collaborating with Hardware Engineers
Close collaboration between software and hardware engineers is crucial for successful integration. Regular communication helps align software requirements with hardware capabilities and resolves potential conflicts early. Collaborative problem-solving and mutual understanding of both disciplines lead to more effective and efficient solutions.
Testing Hardware-Software Interfaces
Testing the interfaces between software and hardware is essential to verify correct operation. Developers use various testing techniques to simulate real-world conditions and validate the integration. Automated testing frameworks and hardware-in-the-loop simulations provide valuable feedback for refining the interfaces and ensuring robust performance.
Keeping Up with Technology
The field of embedded systems is continually evolving, with new hardware and software technologies emerging regularly. Developers need to stay updated with the latest trends and tools to remain effective in their roles.
Embracing Technological Advancements
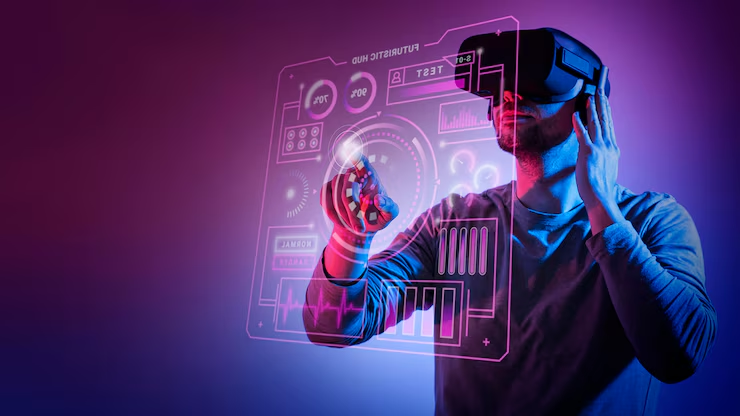
Staying current with technological advancements allows developers to leverage new tools and techniques that enhance productivity and performance. This involves attending industry conferences, participating in training programs, and engaging with the developer community. Embracing innovation helps developers stay competitive and meet evolving industry demands.
Evaluating New Tools and Technologies
Evaluating new tools and technologies involves assessing their potential impact on existing processes and projects. Developers should consider the benefits, risks, and integration challenges associated with adopting new solutions. Thorough evaluation ensures that new technologies align with project goals and enhance overall development efficiency.
Continuous Learning and Skill Development
Continuous learning and skill development are essential for navigating the dynamic landscape of embedded systems. Developers should seek opportunities for professional growth, such as online courses, workshops, and certifications. By investing in their skills, developers can adapt to changing technologies and drive innovation within their organizations.
Conclusion
Embedded software development is a complex but rewarding field that requires careful planning and execution. By following best practices such as clear specification, modular design, thorough testing, and robust documentation, you can develop reliable and efficient embedded software. As technology continues to advance, staying informed and adaptable is key to success in this dynamic industry. Embracing a culture of continuous improvement and collaboration will enable developers to overcome challenges and drive innovation in embedded systems development.
Komentar