Essential Skills for Ruby on Rails Developers
- Staff Desk
- 1 day ago
- 7 min read
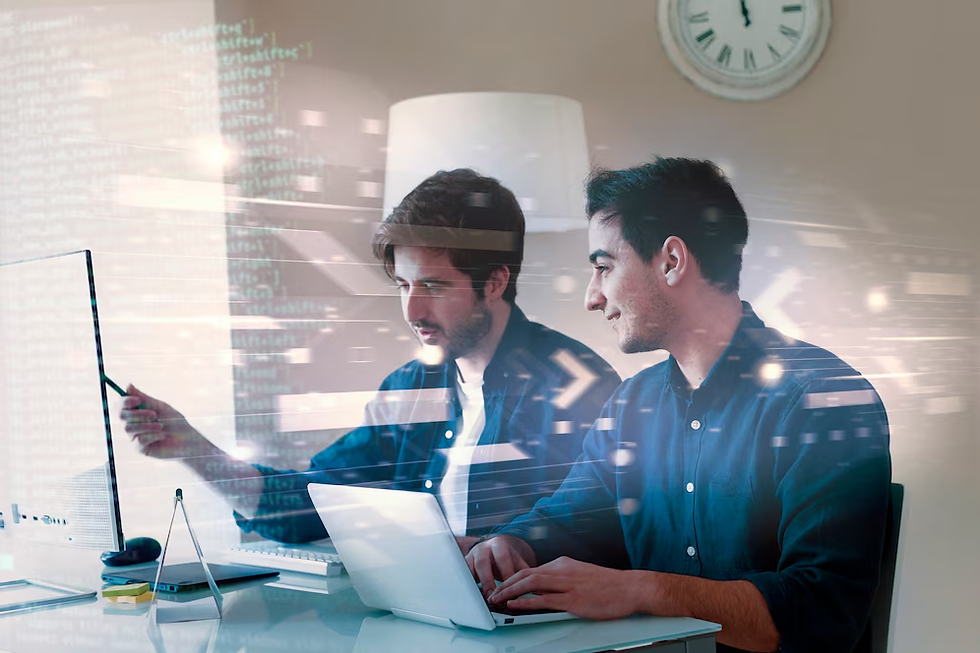
Before diving into Rails, it's crucial to lay a solid foundation in Ruby itself. Ruby is a dynamic, open-source programming language with a focus on simplicity and productivity. Its elegant syntax makes it relatively easy to read and write, which is why it’s favored by developers worldwide. Mastering Ruby sets the stage for a smoother transition into the Rails framework.
Key Ruby Concepts
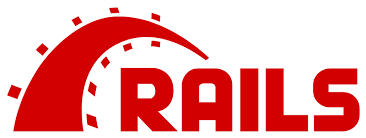
Variables and Data Types
Understanding variables and data types is fundamental in Ruby. Variables are containers for storing data values, and Ruby’s dynamic nature means you can easily manipulate these values. Familiarity with basic data types like strings, numbers, hashes, and arrays is essential. Ruby’s flexibility with data types allows developers to write clean and efficient code, making it crucial to understand how each type operates within the language.
Control Structures
Control structures in Ruby, such as loops, conditionals, and iterators, are vital for controlling the flow of your programs. Loops allow you to repeat actions efficiently, while conditionals enable decision-making within your code. Iterators provide powerful ways to traverse data structures, enhancing your ability to manipulate collections. Grasping these concepts will allow you to write more dynamic and responsive code.
Object-Oriented Programming
Ruby is an object-oriented language, meaning it's centered around objects and classes. You should be comfortable with creating and manipulating classes and objects, understanding inheritance, and utilizing modules. These concepts are not just theoretical; they are practical tools that allow you to build scalable and maintainable applications. Object-oriented programming in Ruby helps manage complexity by organizing code into manageable sections.
Mastering the Rails Framework
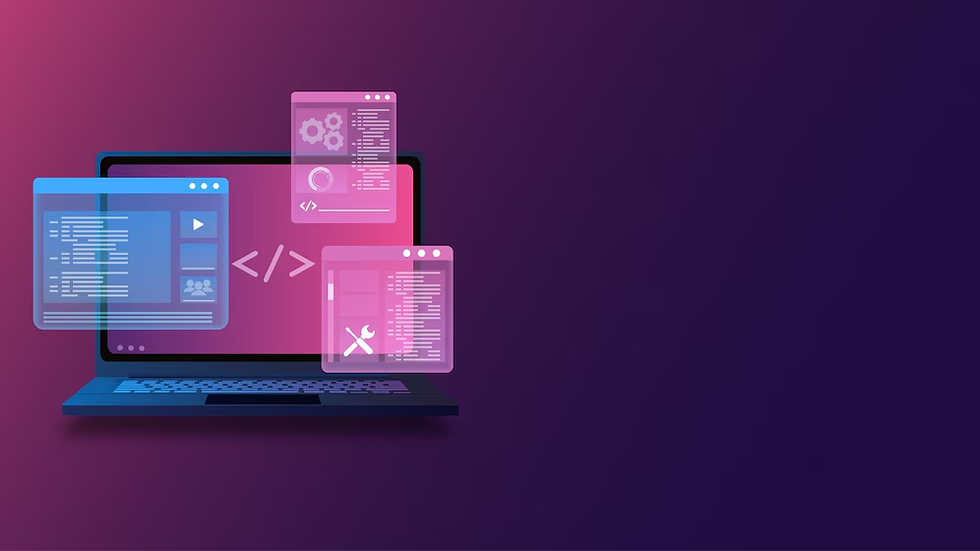
Once you have a handle on Ruby, the next step is to dive into the Rails framework itself. Rails is designed to make programming web applications easier by making assumptions about what every developer needs to get started. This convention over configuration approach allows developers to focus on writing code rather than setting up configurations.
Key Rails Concepts
MVC Architecture
The Model-View-Controller (MVC) architecture is at the heart of Rails. It separates an application into three interconnected components, promoting organized and scalable development. The model handles data and business logic, the view manages user interface and presentation, and the controller processes user input. Understanding this structure is vital for developing organized applications that are easy to maintain and expand.
Routing
Routing in Rails is how incoming web requests are directed to the appropriate controller actions. It’s a crucial part of the Rails framework as it defines the pathways through which users interact with your application. Learning how to configure routes will enable you to create intuitive and user-friendly web applications. Mastering routing ensures that your application can handle user requests efficiently and securely.
ActiveRecord
ActiveRecord is Rails' way of handling database interactions, providing a powerful and flexible interface for database management. It enables you to create, read, update, and delete records in your database with ease. Understanding ActiveRecord is crucial for efficient data handling and retrieval, making it easier to maintain data integrity and streamline database operations. It abstracts complex SQL queries, allowing developers to focus on application logic.
Version Control Systems
Using a version control system like Git is essential for any developer. It allows you to track changes in your code, collaborate with others, and manage different versions of your projects. Version control is a cornerstone of effective software development, ensuring that your work is organized and recoverable.
Git Basics
Repositories
Repositories are the backbone of Git, serving as storage locations for your projects. Understanding how to create and manage repositories is essential for organizing your codebase. Repositories allow you to keep track of every change made to your projects, providing a comprehensive history that can be referenced and restored at any time.
Branching and Merging
Branching and merging are powerful features of Git that allow you to work on multiple features or fixes simultaneously. By creating branches, you can isolate your work, experiment without affecting the main codebase, and then merge successful changes. This practice enhances collaboration and reduces the risk of introducing bugs into the main project.
Commit and Push
Committing changes locally and pushing them to a remote repository, such as GitHub, is a fundamental practice in version control. These actions ensure that your progress is saved and shared with others. Understanding how to effectively commit and push changes will enhance your ability to collaborate and contribute to larger projects efficiently.
Testing and Debugging
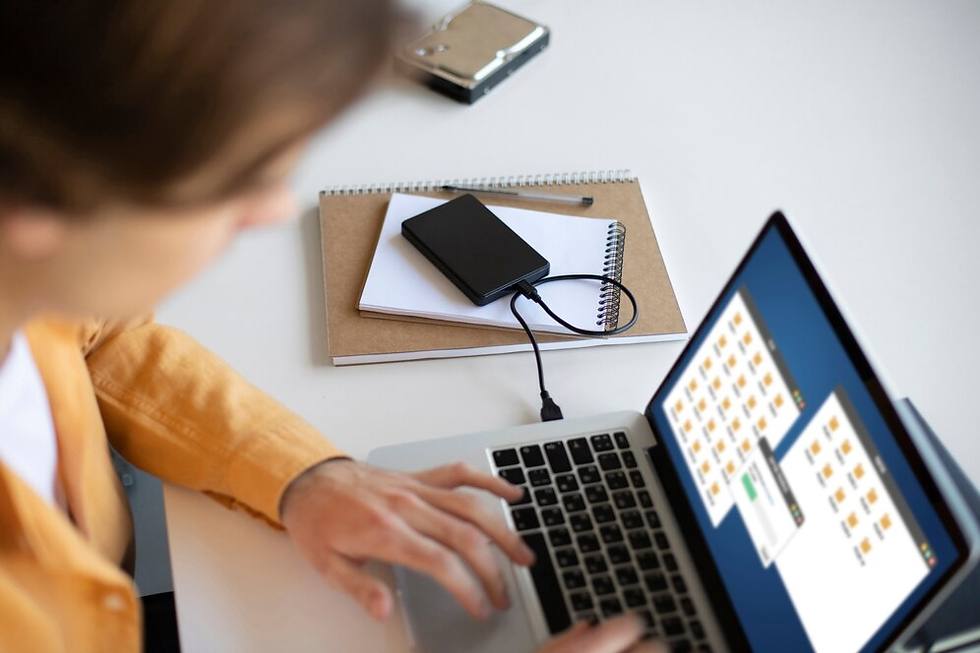
Testing is a critical part of the development process. It ensures that your code works as expected and helps prevent future bugs. Rails has built-in testing support, but there are also other popular testing frameworks like RSpec that offer more advanced capabilities. Testing is not just about finding bugs; it's about ensuring code quality and reliability.
Testing in Rails
Unit Testing
Unit testing involves testing individual components of your application for correct behavior. It focuses on small sections of code, ensuring that each function performs as intended. Unit tests are quick to run and provide immediate feedback, making them invaluable for maintaining code quality throughout development.
Integration Testing
Integration testing ensures that different parts of your application work together as expected. Unlike unit testing, integration tests cover multiple components, validating the interactions between them. This type of testing helps catch issues that may arise from the integration of various parts of your application, ensuring a seamless user experience.
Test-Driven Development (TDD)
Test-Driven Development (TDD) is a practice where tests are written before the code they validate. This approach leads to more reliable code by forcing developers to consider edge cases and requirements upfront. TDD promotes a deeper understanding of the problem domain and results in a more robust application architecture.
Front-End Development Skills
While Rails handles the back-end logic, a good developer should also be familiar with front-end technologies. This knowledge enables you to create more interactive and user-friendly applications. Understanding front-end development is essential for delivering a complete web application experience.
Front-End Basics
HTML & CSS
HTML and CSS are the building blocks of web development. HTML structures your web pages, while CSS styles them, creating visually appealing interfaces. Mastery of these technologies is essential for any developer looking to create responsive and accessible web applications.
JavaScript
JavaScript adds interactivity to your Rails applications, allowing you to create dynamic user experiences. A basic understanding of JavaScript is crucial for implementing features like form validation, dynamic content updates, and asynchronous requests. This knowledge can significantly enhance the functionality and user engagement of your web applications.
Responsive Design
Responsive design ensures that your applications work well on a variety of devices and screen sizes. Understanding how to create flexible layouts and adapt content to different screens is critical in today's multi-device world. Responsive design principles allow you to provide a consistent user experience across desktops, tablets, and smartphones.
Deployment and Hosting
Understanding how to deploy and host your Rails applications is another essential skill. There are various hosting services available, such as Heroku, AWS, and DigitalOcean, which can simplify the deployment process. Deployment is the final step in making your application available to users, and mastering it ensures a smooth transition from development to production.
Deployment Essentials
Environment Configuration
Managing different environments (development, test, production) and configuring them accordingly is crucial for deployment. Each environment has unique requirements and configurations, and understanding these differences ensures that your application runs smoothly in all scenarios. Proper environment configuration can prevent many common deployment issues.
Continuous Integration/Continuous Deployment (CI/CD)
CI/CD tools automate the testing and deployment process, ensuring that new code can be integrated seamlessly. Familiarizing yourself with these tools can greatly enhance your development workflow, reducing errors and speeding up delivery. CI/CD practices promote a culture of continuous improvement and collaboration within development teams.
Soft Skills for Ruby on Rails Developers
Technical skills are only part of the equation. Soft skills are just as important in the tech industry and can significantly impact your success as a developer. They enhance your ability to work effectively within a team and adapt to the ever-changing technology landscape.
Important Soft Skills
Problem-Solving
Problem-solving is the ability to approach and solve complex problems efficiently. This skill is crucial for debugging, optimizing code, and developing innovative solutions. A strong problem-solving mindset enables developers to tackle challenges head-on and deliver high-quality software.
Communication
Effective communication is key to collaborating with team members and articulating your ideas clearly. Whether you're discussing project requirements or providing feedback, strong communication skills facilitate better teamwork and project outcomes. Good communication helps bridge the gap between technical and non-technical stakeholders.
Adaptability
Adaptability is the willingness to learn new technologies and adapt to changes in the industry. The tech world is constantly evolving, and staying open to new ideas and methodologies is essential for long-term success. An adaptable developer can quickly integrate new tools and practices, staying ahead in the competitive field of web development.
Staying Updated with the Rails Community
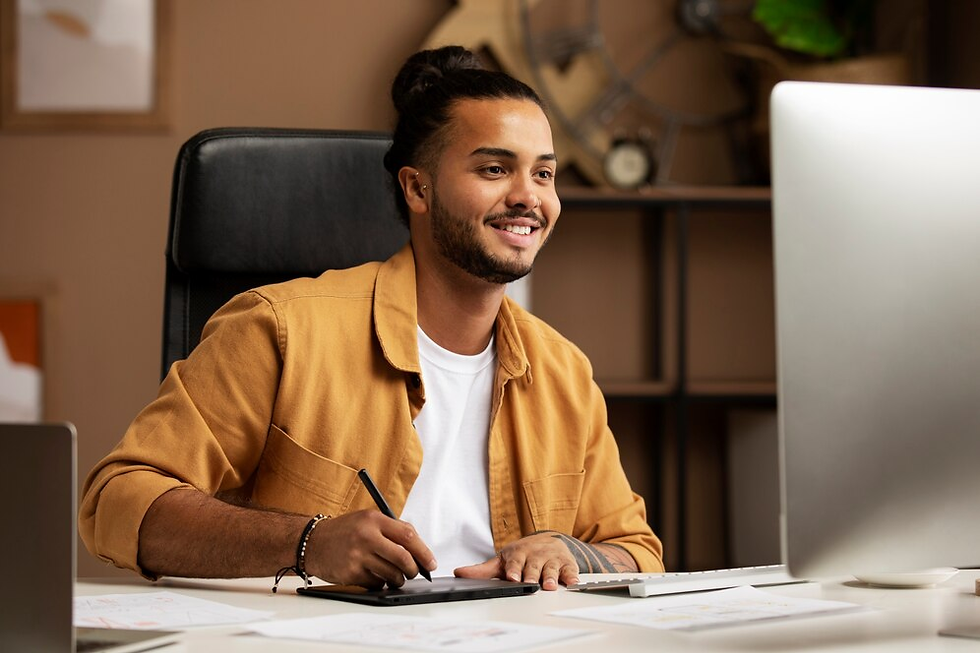
Technology is always evolving, and staying updated with the latest trends and practices is crucial. Engaging with the Rails community can provide valuable insights and learning opportunities. Community involvement can also lead to professional growth and networking opportunities.
Community Engagement
Online Forums and Meetups
Participating in online forums like Stack Overflow or attending local meetups and conferences can greatly enhance your knowledge and network. These platforms provide a space to ask questions, share knowledge, and connect with other developers. Engaging in these communities can lead to collaborative opportunities and career advancements.
Open Source Contributions
Contributing to open-source projects is a fantastic way to improve your skills and connect with other developers. Open source involvement allows you to work on real-world projects, receive feedback from experienced developers, and contribute to the community. It’s an excellent way to showcase your skills and dedication to potential employers.
Conclusion
Mastering Ruby on Rails requires a blend of technical knowledge, practical experience, and continuous learning. By focusing on these essential skills and engaging with the community, you'll be well-equipped to build dynamic and efficient web applications. Whether you're a beginner or a seasoned developer, refining these skills will help you advance in your career as a Ruby on Rails developer. Embrace the journey of learning and improvement, and you'll find success in the ever-evolving world of web development.
Comments